Inheritance
When we say, “a student IS-A person”, “an employee IS-A person” “a car IS-A vehicle”, “a bus IS-A vehicle”, etc. This indicates that student and employee share some common attributes and behaviors, the same for car and bus.
Inheritance provides a generic super class, an abstract class, that has the common attributes and methods between all sub classes.
This way, we avoid duplicates, as we don’t have to write the common attributes or methods. You only write them once in the super class, and they automatically will have all the attributes and methods from their super class.
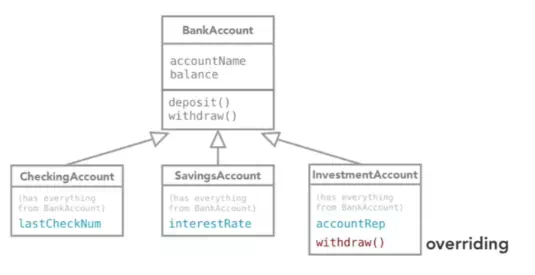
We may add some methods, or attributes that are specific to the sub class.
Something to watch out for, if you want to re-write the inherited methods in the sub class, we’re doing what’s called overriding.
Overriding is replacing or adding additional behavior to the inherited one.
This is a not good case practice here, unless you have an abstract method in the super class. The abstract methods will be explained later.
Another thing is, try to avoid the situation where you have many level of inheritance — Depth of Inheritance (DIT). Don’t look for inheritance, inheritance announces for it self.
Association, Aggregation and Composition
We have been identifying relationships between objects by drawing a line between any two objects in our conceptual model.
This may indicates an association relationship. It means that an object has to know about, or, interact with another object(s). It might be for example, a customer object needs to call a method of shopping cart.
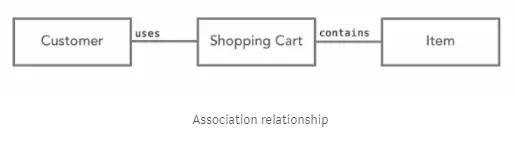
In other words, take a look at the following code snippet.
It shows that customer object uses a shopping cart object to get the number of items in the customer’s cart, and the shopping cart object needs to use an itemobject to add items to the cart.
public class Customer {
private ShoppingCart myCart;
// …
public int items(){
return myCart.getItemCount();
}
}
public class ShoppingCart {
private ArrayList items;
// …
public void addItem(Item t) {
items.add(t);
}
public int getItemCount() {
return items.size();
}
}
Aggregation relationship is also association. But, it describes that an object can be made of, or built from some other objects.
For example, “a car HAS-A engine”, “a classroom HAS-A student(s)”, etc. It refers to the “HAS-A” relationship rather than the “IS-A” relationship as in inheritance. So, we can’t say “a car IS-A seat”.
Composition is the same as aggregation, but it implies the concept of ownership. Thus, it’s a more specific form of aggregation.
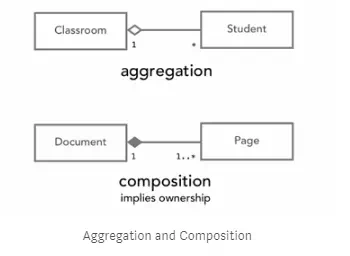
For example, if we have a document class and page class, and, the documentobject is composed of, made of page object(s).
If at anytime the document object hasn’t been instantiated yet; it doesn’t exist, the page object shouldn’t also be exist.
Similarly, If we destroyed the document object, then the associated page object(s) should be destroyed too. It can’t exist logically unless the documentobject exists.
Now, when to use aggregation vs composition?. Use aggregation when the objects can exist independently, and use composition if these objects can’t logically exist without that object that’s made of them.