An array is a data structure for storing more than one data item that has a similar data type. The items of an array are allocated at adjacent memory locations. These memory locations are called elements of that array.
The total number of elements in an array is called length. The details of an array are accessed about its position. This reference is called index or subscript.
Concept of Array
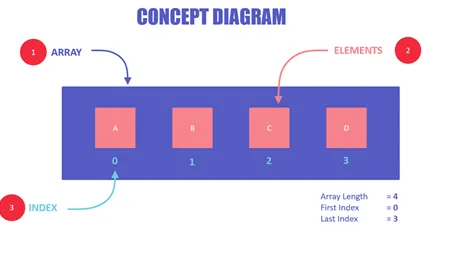
Concept Diagram of Arrays
The above diagram illustrates that:
- An array is a container of elements.
- Elements have a specific value and data type, like “ABC”, TRUE or FALSE, etc.
- Each element also has its own index, which is used to access the element.
Note:
- Elements are stored at contiguous memory locations.
- An index is always less than the total number of array items.
- In terms of syntax, any variable that is declared as an array can store multiple values.
- Almost all languages have the same comprehension of arrays but have different ways of declaring and initializing them.
- However, three parts will always remain common in all the initializations, i.e., array name, elements, and the data type of elements.
The following diagram illustrates the syntax of declaring an array in Python and C++ to present that the comprehension remains the same though the syntax may slightly vary in different languages.
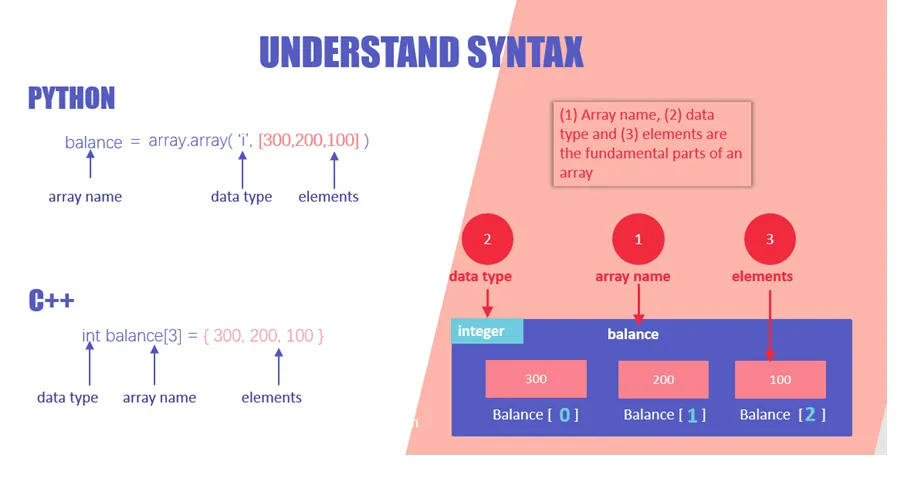
Understand Syntax of Arrays
- Array name: necessary for easy reference to the collection of elements
- Data Type: necessary for type checking and data integrity
- Elements: these are the data values present in an array
Why do we need arrays?
Here, are some reasons for using arrays:
- Arrays are best for storing multiple values in a single variable
- Arrays are better at processing many values easily and quickly
- Sorting and searching the values is easier in arrays
Creating an Array in Python
In Python, arrays are different from lists; lists can have array items of data types, whereas arrays can only have items of the same data type.
Python has a separate module for handling arrays called array, which you need to import before you start working on them.
Note: The array must contain real numbers like integers and floats, no strings allowed.
The following code illustrates how you can create an integer array in python to store account balance:
import array
balance = array.array('i', [300,200,100])
print(balance)
Ways to Declare an Array in Python
You can declare an array in Python while initializing it using the following syntax.
arrayName = array.array(type code for data type, [array,items])
The following image explains the syntax.
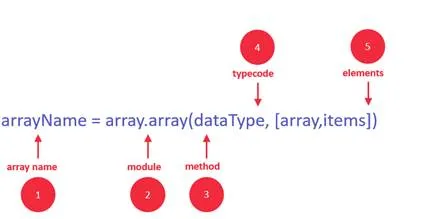
Syntax of Array in Python
- Identifier: specify a name like usually, you do for variables
- Module: Python has a special module for creating arrays, called “array” – you must import it before using it
- Method: the array module has a method for initializing the array. It takes two arguments, typecode, and elements.
- Type Code: specify the data type using the typecodes available (see list below)
- Elements: specify the array elements within the square brackets, for example [130,450,103]
The following table illustrates the typecodes available for supported data types:
Type code | C Type | Python Type | Minimum size in bytes |
‘c’ | char | character | 1 |
‘B’ | unsigned char | int | 1 |
‘b’ | signed char | int | 1 |
‘u’ | Py_UNICODE | Unicode character | 2 |
‘h’ | signed short | int | 2 |
‘H’ | unsigned short | int | 2 |
‘i’ | signed int | int | 2 |
‘I’ | unsigned int | long | 2 |
‘l’ | signed long | int | 4 |
‘L’ | unsigned long | long | 4 |
‘f’ | float | float | 4 |
‘d’ | double | float | 8 |
How to access a specific array value?
You can access any array item by using its index.
SYNTAX
arrayName[indexNum]
EXAMPLE
balance[1]
The following image illustrates the basic concept of accessing arrays items by their index.
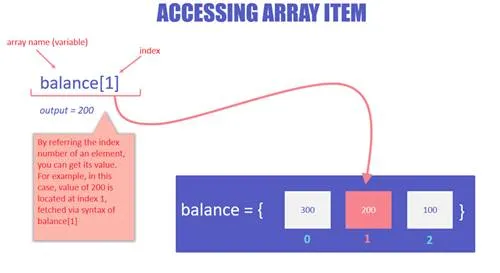
Access an Array Element
Here, we have accessed the second value of the array using its index, which is 1. The output of this will be 200, which is basically the second value of the balanced array.
import array
balance = array.array('i', [300,200,100])
print(balance[1])
OUTPUT
https://tpc.googlesyndication.com/safeframe/1-0-37/html/container.html 200
Comments are closed.