An abstract class is a class that can’t be instantiated; can’t create objects from it. It exists only for being inherited.
It exists to provide a generic class, that has some common attributes, and methods shared across all inherited classes, and you will never instantiate an object from that class. On the other side, a concrete class can be instantiated.
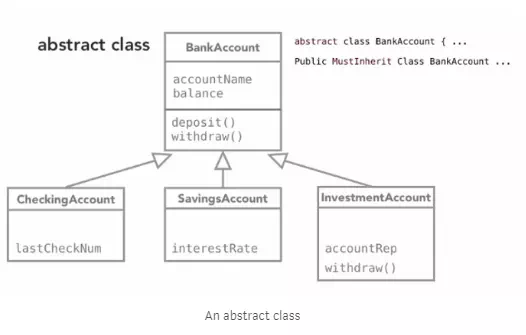
We mark a class to be abstract by using the keyword “abstract” in the class declaration.
abstract public class BankAccount {
// your code goes here
}
In abstract class, methods and attributes don’t have to be static, so that they can be accessible by any object of a class that inherit from that abstract class.
Abstract Methods
An abstract method is a method that is declared without an implementation, and it must be inside an abstract class.
All sub classes must override the abstract methods. This is the case where overriding the inherited methods is not only allowed, but it’s mandatory.
abstract public class BankAccount {
abstract public void withdraw();
}
In the abstract class, we can also have non-abstract methods that are shared across all the sub classes.
Interfaces
It’s an abstract class implicitly (you don’t write the keyword “abstract)”, but, it consists of a list of methods.
It exists also for being inherited, and overridden by the sub classes.
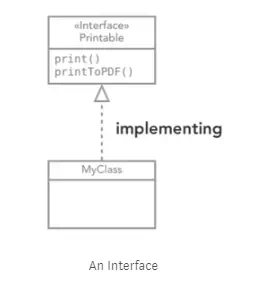
public interface Printable {
public void print();
public void printToPDF();
}
An important question that must be raised here, what should we use when we have an inheritance situation, a concrete class or an interface?. Well, It depends on what you want to do:
· If the common methods and attributes shared between all sub classes, you need a concrete class, so you write the code only once, and the sub classes will have it by default.
· If, and on the other hand, there are common methods between sub classes, but, each sub class has it’s own different implementation, we need an interface.
It’s true that many developer’s prefer using interface is to provide formal list of methods to support rather than inheriting from a concrete class and dealing with lots of pre-provided functionality that may or may not be correct.
Comments are closed